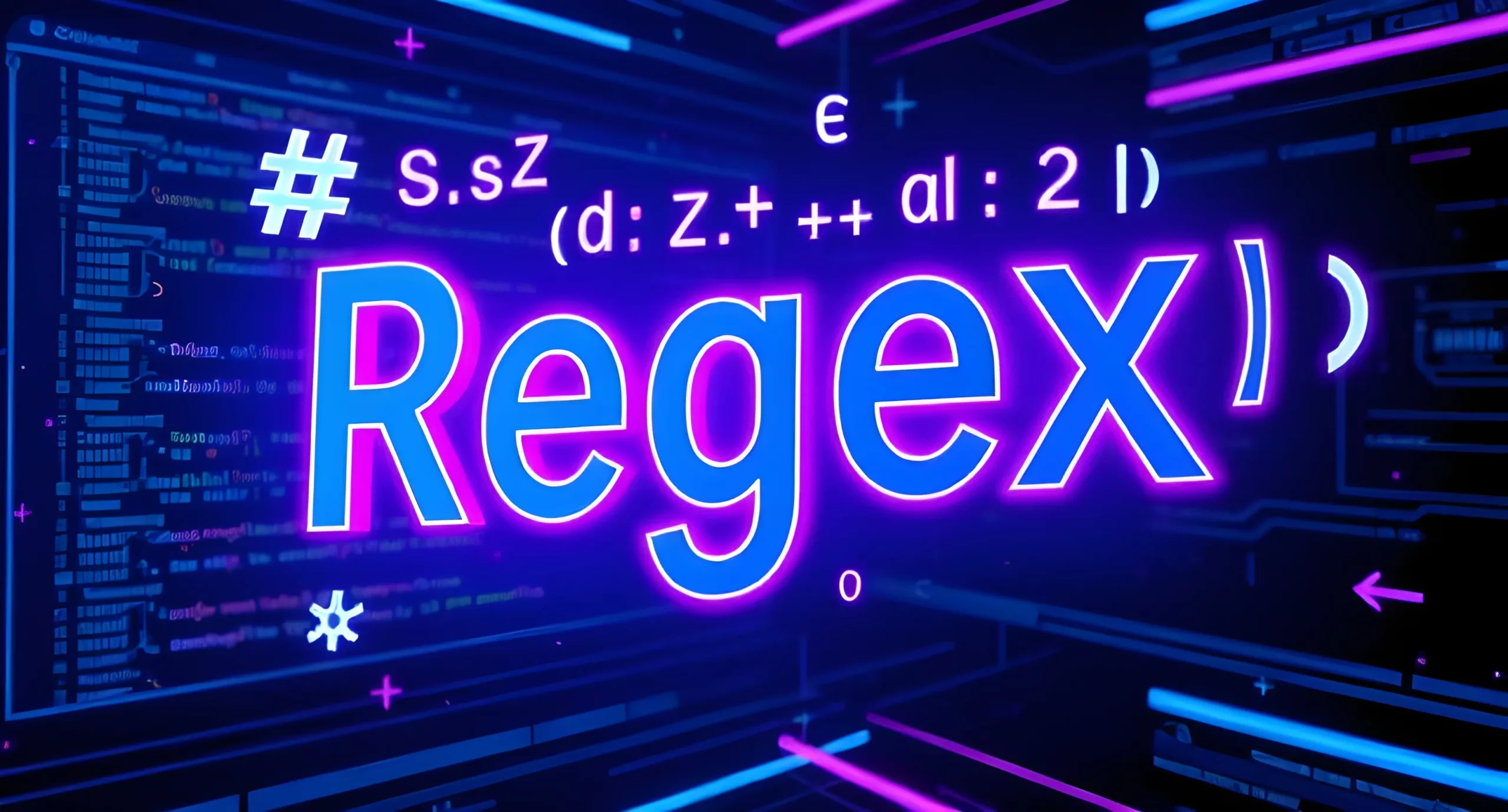
When searching for a fixed word, you just type it in. But what if you need to find dynamic text like emails, crypto wallet addresses, URLs, trading signals, transaction data, or even hidden patterns—without knowing the exact string? This is where Regex (Regular Expressions) becomes a game-change ❤️
Regex (Regular Expressions) is the ultimate solution! This powerful tool allows you to search, replace, and validate text efficiently, making it essential for programming, text processing, automation, and even financial data filtering.
How Regex Works
A regular expression consists of special characters used to match one or more text patterns. It operates based on both normal and special characters.
Examples:
abc
matches exactly “abc”.[a-z]
matches any lowercase letter from “a” to “z”.\d
matches any digit from 0 to 9.hello|world
matches either “hello” or “world”.(abc)+
matches “abc”, “abcabc”, “abcabcabc”.
Special Characters in Regex
Basic Character Groups
Character | Meaning |
---|---|
. | Matches any character except newline |
^ | Matches the beginning of a string |
$ | Matches the end of a string |
* | Matches the preceding character zero or more times |
+ | Matches the preceding character at least once |
? | Matches the preceding character zero or one time |
{n} | Matches exactly n occurrences of the preceding character |
{n,} | Matches at least n occurrences |
{n,m} | Matches between n and m occurrences |
Examples:
a*
matches “”, “a”, “aa”, “aaa”, etc.a+
matches “a”, “aa”, “aaa” but not “”.a{2,4}
matches “aa”, “aaa”, “aaaa”, but not “a” or “aaaaa”.colou?r
matches both “color” and “colour”.
Regex – Wildcard Character Groups
Character | Meaning |
\d | Matches any digit (0-9) |
\D | Matches any non-digit character |
\w | Matches any alphanumeric character (a-z, A-Z, 0-9, _) |
\W | Matches any non-alphanumeric character |
\s | Matches whitespace (space, tab, newline) |
\S | Matches any non-whitespace character |
Examples:
\d{3}
matches “123”, “456” but not “12” or “1234”.\w+
matches “hello”, “abc123” but not “@!#”.\s+
matches any whitespace between words.
Grouping and Alternation in Regex
Using Parentheses ()
Parentheses ()
allow grouping of multiple characters to apply operators such as +
, *
, ?
, {n,m}
.
Examples:
(ab)+
matches “ab”, “abab”, “ababab” but not “a” or “b”.(\d{2}-){2}\d{4}
matches “12-34-5678”.
Using |
for OR
The pipe |
is used to select one option from multiple choices.
Examples:
cat|dog
matches either “cat” or “dog”.a(b|c)d
matches “abd” or “acd”.(jpg|png|gif)$
matches image file extensions.
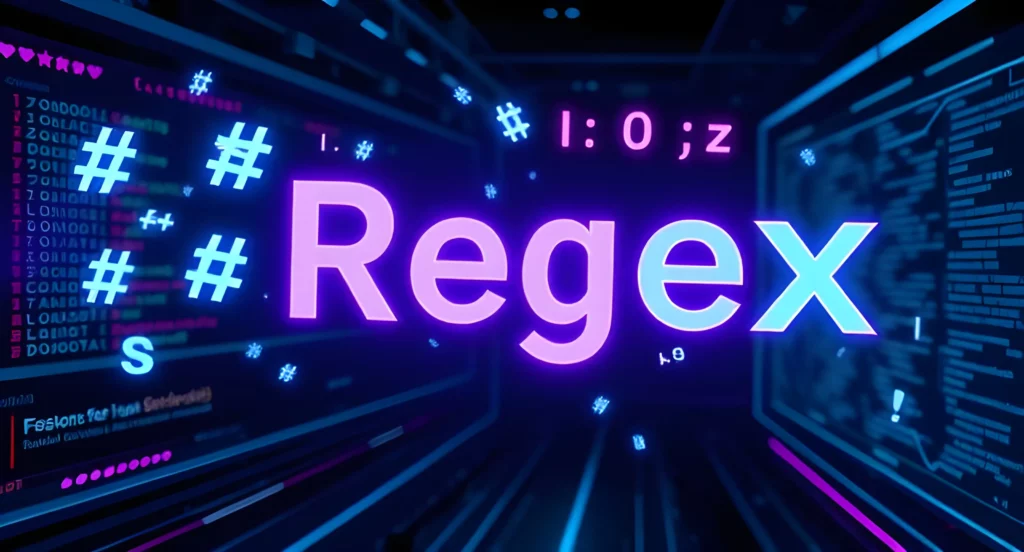
Practical Applications of Regex
Note: if you have used a language translator for this article, please temporarily switch to English to see the most accurate example.
1. Replace Email
Original Text: For support, email us at [email protected]
Replace with: [email protected]
Result: For support, email us at [email protected]
2. Replace URLs
Original Text: Visit our website at https://example.com for more details.
Replace with: you_link
Result: Visit our website at you_link.com for more details.
3. Formatting Cryptocurrency Prices
Original Text: BTC is currently trading at 456789.235 USDT.
Replace with: \1,
Result: BTC is currently trading at 456,789.235 USDT.
4. Masking Specific Price Levels
Original Text: BTC has strong resistance at 42000 and support at 35000.
Replace with: XXXXX
Result: BTC has strong resistance at XXXXX and support at 35000.
5. Hiding Stop-Loss (SL) Values
Original Text: SL: 39500, TP: 45000.
Replace with: SL: XXXX
Result: SL: XXXX, TP: 45000.
5. The salvage value (Liquidation Price)
Original Text: Liquidation Price: 31250 USDT.
Replace with: HIDDEN
Result: HIDDEN
6. Trading Volume Data
Original Text: Trading Volume: 2.3 BTC today.
Replace with: Volume: X COIN
Result: Volume: X COIN today.
7. Replace the important event in the Crypto market
Original Text: FOMC meeting is coming up, expect volatility.
Replace with: MARKET_EVENT
Result: MARKET_EVENT meeting is coming up, expect volatility.
8. Replace the new token listing announcement
Original Text: New Listing: XYZ token now available on Binance!
Replace with: NEW_TOKEN_ALERT
Result: NEW_TOKEN_ALERT: XYZ token now available on Binance!
9. Replace the Entry price in the transaction
Original Text: Entry Price: 41250 USDT on BTC/USDT pair.
Replace with: Entry Price: XXXX
Result: Entry Price: XXXX on BTC/USDT pair.
10. Replace the Exit price in the transaction
Original Text: Exit Price: 43500 USDT with 10% profit.
Replace with: Exit Price: XXXX
Result: Exit Price: XXXX with 10% profit.
11. Replace the order status (Open/Closed)
Original Text: Trade Status: Open, waiting for TP.
Replace with: STATUS
Result: Trade Status: STATUS, waiting for TP.
12. Wallet Addresses (Ethereum, BSC, BEP-20, Polygon, etc…)
Original Text: Send USDT to 0x4aF3D1e2b9F8c4567d7eB123FabcDe45a6D7B92c now!
Replace with: you_address
Result: Send USDT to you_address now!
13. Replace Bitcoin Addresses
Original Text: Send BTC to 1A1zP1eP5QGefi2DMPTfTL5SLmv7DivfNa
Replace with: you_address
Result: BTC Send BTC to you_address
14. Replace Solana Wallet Addresses
Original Text: SOL wallet: 8fj4p2HL1kLx7SDFjWLxqJ5BFsGHTAPmXKtK8J9J8xrb
Replace with: you_address
Result: SOL wallet: you_address
15. Replace TRON (TRX, USDT-TRC20) Wallet Addresses
Original Text: TRX Wallet: TMaMZGMNwD9cFSXvTzSjqZ6v7YyLRMnM8k
Replace with: you_address
Result: TRX Wallet: you_address .
16. Replace XRP Wallet Addresses
Original Text: XRP Address: rDsbeomae4FXwgQTJp9Rs64Qg9vDiTCdBv
Replace with: you_address
Result: XRP Address: you_address
17. Change good or perfect to bad
Original Text: This is a good and perfect example.
Replace with: bad
Result: This is a bad and bad example.
18. Remove “✉️TPA trading report:”, “TPA: Entry”, “The monitoring will be continued.”
Original Text:
✉️TPA trading report:
TPA: Entry BUY UUDCHF M30 at 2023.06.16 15:30
The monitoring will be continued.
Replace with: \1\2
Result: BUY UUDCHF M30 at 2023.06.16 15:30
19. Change all “Take profit (1|2|3)👉at” to TP
Original Text:
🚨Signal Alert🚨
GOLD sell at (@ 1966.40)
Take profit 1👉at 1955.35
Take profit 2👉at 1938.94
Take profit 3👉at 1918.62
Stop loss at 1982.45 🎯
Chance of success: 83%
⚠️Risk 1-2% per trade!
Replace with: TP
Result:
🚨Signal Alert🚨
GOLD sell at (@ 1966.40)
TP 1955.35
TP 1938.94
TP 1918.62
Stop loss at 1982.45
🎯Chance of success: 83%
⚠️Risk 1-2% per trade!
20. Change all to new format
Original Text:
🔥 #RDNT/USDT (Long📈, x20) 🔥
Entry – 0.2483
SL – 25-30%
Take-Profit:
🥇 0.2534 (40% of profit)
🥈 0.256 (60% of profit)
🥉 0.2586 (80% of profit)
🚀 0.2614 (100% of profit)
Replace with: \1 Binance futures LEVERAGE CROSS \ BUY \3 SELL: \5 \6 \7 \8 STOP: \4%
Result:
RDNTUSDT Binance futures
LEVERAGE CROSS 20X
BUY 0.2483
SELL: 0.2534 0.256 0.2586 0.2614
STOP: 25%
Conclusion
Regex is an extremely useful tool for flexible and efficient text processing. Mastering it can significantly improve your ability to manage data, validate inputs, and work with textual content professionally.
Furthermore, if you need to use Regex for automated message forwarding, this Bot is the ideal choice 👉 Click here
👉 See More: How to Create Automatic Message Forwarding in Telegram